How to write rest API in GO
Go is a statically typed language developed by google in 2007.
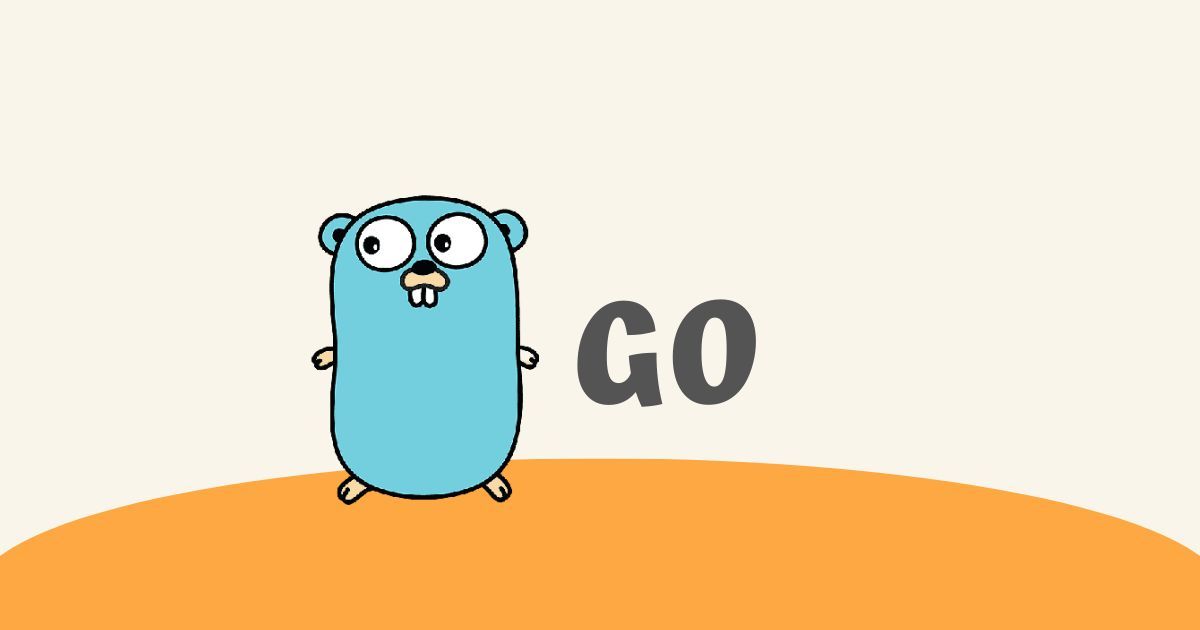
Go is a statically typed language developed by google in 2007. This implies that in GO once a variable has been declared of a certain type it cannot be changed letter. i.e In GO you cannot change an integer to a string if the variable was declared as an integer however this is possible in Python a dynamically typed language.
Statically typed languages check for objects types at compile time while dynamically typed languages such as Python check for object types at runtime.
Go syntax is similar to C however it comes with memory safety and garbage collection inbuilt.
Over the years Go has be used with success in many open source projects such Traefik, Kubernetes,Hugo and many more. Go main key take away being speed and its ease in running tasks concurrently.
Installing Go:
Before getting started we need to have go installed our system. Depending on your operating system use the Go installation guide to install Go.
If your using a Linux system you can run the following script as root
wget -c https://dl.google.com/go/go1.19.4.linux-amd64.tar.gz
rm -rf /usr/local/go && tar -C /usr/local -xzf go1.19.4.linux-amd64.tar.gz
export PATH=$PATH:/usr/local/go/bin
chmod u+x install-go.sh
the run it as sudo .\install-go.sh
Getting started with GO
We'll start by creating our directory.
mkdir go-rest-api && cd go-rest-api
Next we'll initialize go modules dependency tracking.
go mod init github.com/<github-username>/go-rest-api
This creates a go.mod
file which is used for tracking our dependencies.
We then need to add the gin
go package to our packages. Gin is a go module that enables Go to serve http requests. Run the below command.
go get -u github.com/gin-gonic/gin
Now that we have all our dependencies we can start writing code.
Create a file main.go
Now lets write a simple Go rest API in main.go
.
package main
import (
"net/http"
"github.com/gin-gonic/gin"
)
// album represents data about a record album.
type album struct {
ID string `json:"id"`
Title string `json:"title"`
Artist string `json:"artist"`
Price float64 `json:"price"`
}
// albums slice to seed record album data.
var albums = []album{
{ID: "1", Title: "Blue Train", Artist: "John Coltrane", Price: 56.99},
{ID: "2", Title: "Jeru", Artist: "Gerry Mulligan", Price: 17.99},
{ID: "3", Title: "Sarah Vaughan and Clifford Brown", Artist: "Sarah Vaughan", Price: 39.99},
}
func main() {
router := gin.Default()
router.GET("/albums", getAlbums)
router.GET("/albums/:id", getAlbumByID)
router.POST("/albums", postAlbums)
router.Run("localhost:8080")
}
// getAlbums responds with the list of all albums as JSON.
func getAlbums(c *gin.Context) {
c.IndentedJSON(http.StatusOK, albums)
}
// postAlbums adds an album from JSON received in the request body.
func postAlbums(c *gin.Context) {
var newAlbum album
// Call BindJSON to bind the received JSON to
// newAlbum.
if err := c.BindJSON(&newAlbum); err != nil {
return
}
// Add the new album to the slice.
albums = append(albums, newAlbum)
c.IndentedJSON(http.StatusCreated, newAlbum)
}
// getAlbumByID locates the album whose ID value matches the id
// parameter sent by the client, then returns that album as a response.
func getAlbumByID(c *gin.Context) {
id := c.Param("id")
// Loop through the list of albums, looking for
// an album whose ID value matches the parameter.
for _, a := range albums {
if a.ID == id {
c.IndentedJSON(http.StatusOK, a)
return
}
}
c.IndentedJSON(http.StatusNotFound, gin.H{"message": "album not found"})
}
Once you are done you can run go as below:
go run main.go
Resources:
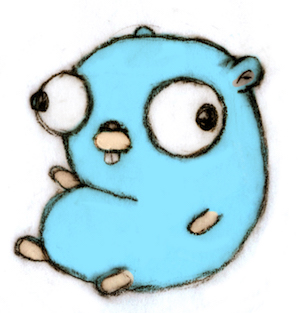