Getting starting with Rust + Actix Web for beginner
Big tech companies such a Google, Microsoft and Netflix use rust. i.e. Google is currently using rust in the development of Android operating system
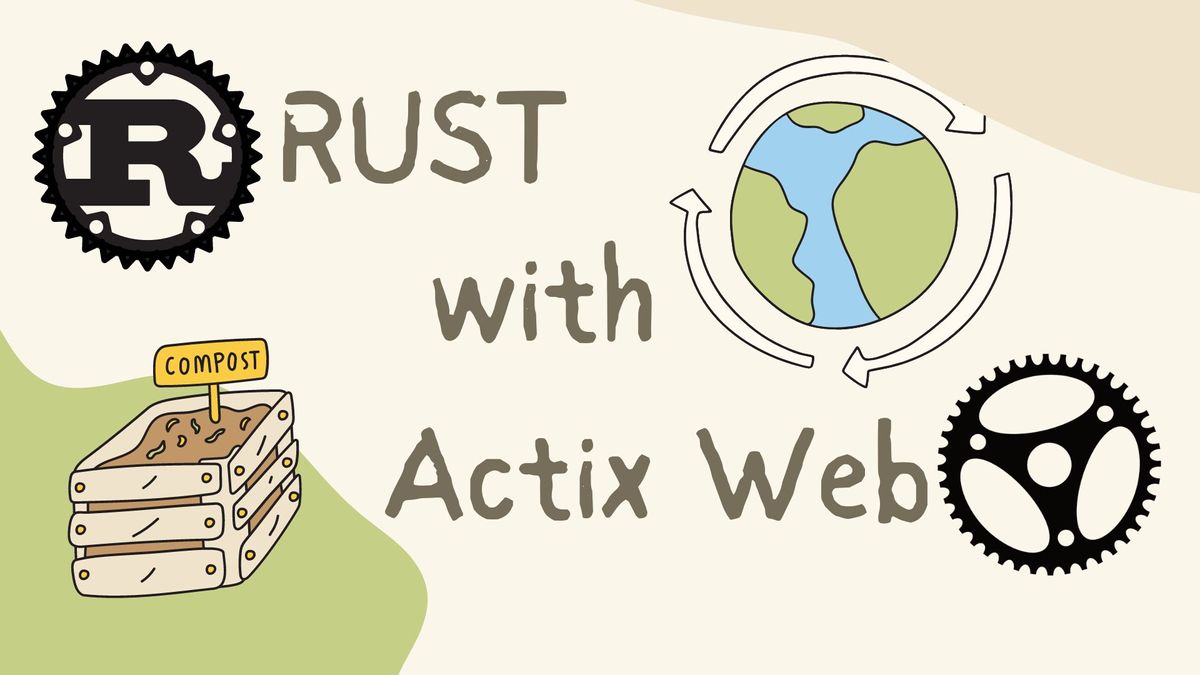
Rust is a low-level programming language developed by Mozilla. Its primal focus is safety, speed, and concurrency. Unlike its predecessors such as C++ and C, rust is considered a more modern low-level programming language. Rust borrows some of its syntax from popular programming languages such as JavaScript to make the development experience more friendly.
Big tech companies such as Google, Microsoft, and Netflix use rust. i.e. Google is currently using rust in the development of the Android operating system. The main reason for using rust in most companies is memory safety. Rust was written with memory safety in mind. Nobody wants to write code that's going to crush on the client or worse riddled with bugs. Code that is usually memory-safe is usually more secure.
Actix Web
Actix web is a micro framework like the python flask framework however its architecture looks more like NodeJS express web framework. Actix web is among the fastest web frameworks out there. Actix web performance is just outstanding this is no surprise because actix web is based on rust a low-level programming language.
Installing Rust
If you use windows, I recommend running rust in Windows Subsystem for Linux.
Run the below command to install rust.
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
curl
installed on your system. On Debian or ubuntu run
sudo apt install curl
this may vary depending on your Linux operating system.After installation, configure your shell by running the below command.
source "$HOME/.cargo/env"
Getting Started with Actix Web
We'll start by creating a directory sample-app
cargo new sample-app
cd sample-app
NB: The sample project structure should look like below.
sample-app
βββ Cargo.toml
βββ src
βββ main.rs
Cargo.toml
Cargo.toml
is the manifest file for rust package manager cargo it is more like package.json
in NodeJS. Rust borrows a lot from modern programming languages. Low-level programming languages like C and C++ do not have a package manager. Cargo.toml
usually contains metadata of the running rust program. The metadata usually includes data such as the name, version, dependencies, etc
We will add Actix Web
as our dependency in the Cargo.toml
. Cargo.toml
should look like the one below.
[package]
name = "sample-app"
version = "0.1.0"
edition = "2021"
[dependencies]
actix-web = "4"
src/main.rs
src/main.rs
this is where we write our main rust code.
Replace the content in src/main.rs
with the code below.
use actix_web::{get, post, web, App, HttpResponse, HttpServer, Responder};
#[get("/")]
async fn hello() -> impl Responder {
HttpResponse::Ok().body("Hello world!")
}
#[post("/echo")]
async fn echo(req_body: String) -> impl Responder {
HttpResponse::Ok().body(req_body)
}
async fn manual_hello() -> impl Responder {
HttpResponse::Ok().body("Hey there!")
}
#[actix_web::main]
async fn main() -> std::io::Result<()> {
HttpServer::new(|| {
App::new()
.service(hello)
.service(echo)
.route("/hey", web::get().to(manual_hello))
})
.bind(("127.0.0.1", 8080))?
.run()
.await
}
If you have ever worked with any web framework the code is easier to understand. We import the necessary modules in the first chunk of code. we then define a get method
function, a post method
and a manual function
that can be bound as a route.
The last chunk of code is Β the main function
where we initialize the methods
as services
and functions
as routes
. We then bind it to the IP and Port we want our actix-web
to be exposed on.
NB: Actix-web runs asynchronously to prevent render blocks
Run Rust/ Actix-web
To run actix-web with rust use Cargo run
Cargo run